[Java 기초문법] by Professional Java Developer Career Starter: Java Foundations @ Udemy
Data types
BYTE (-128 to 127)
아스키코드에서 A가 65에 assgined 된 것을 확인했다.
random하게 된 것이 아니라는 점.
컴퓨터가 글자를 숫자로 인식하는 방식의 가장 lowest level은 bits 단위를 보는 것이다.
8 bits = 1 BYTE
이진법으로 65는
8 7 6 5 4 3 2 1
128 64 32 16 8 4 2 1
0 1 0 0 0 0 0 1
Hexadecimal 에서 FACE는 ?
E = 1110
C = 1100
A = 1010
F = 1111
public class NumberStuff {
public static void main(String[] args) {
byte myByte = 13;
System.out.println(myByte);
13은 @2F3582AF21 과 같은 주소에 저장되는 한편
이진수로 1101로 저장된다.
8 gigs of RAM의 컴퓨터라고 하면 약 8백만 bytes의 공간이 할당되어 있음.
만약 boolean을 할당하면 어떻게 될까?
public static void main(String[] args) {
byte myByte = 13;
boolean myFlag = true;
System.out.println(myFlag);
a bit is allocated, and either be a one or zero.
0 = false
1 = true
by default로서 byte에 저장할 수 있는 largest 는 이진수로 -128 ~ 127이다
=> 10진수로 -255 ~ 255
negative는 어떻게 표현될까?
1 0 0 0 0 0 0 1 <= 129가 아니라 negative 128로 표현됨
Short = 16 bits = 2 bytes = -32,768 to 32,767 => CHAR
그런데 알파벳이 그렇게 많지 않은데 이렇게 많이 할당할 필요가 있을까 ?
to represent all of the lg in the world, not only english or latin
자바의 character system에서 다르게 저장된다.
char myChar = '강';
char myA = 'A'; // in memory 65
A는 ASCII로 65로 직관적으로 이해되지만
Korean은 sillable이 나눠져 있어서 어떻게 계산이 될까?
=> a lot of characters required
=> char => needs thousands and thousands
INT = 32 bits => -2,147,483,648 ~ 2,147,483,647
LONG = almost * 2 INT => -9,223,372,036,854,775,808 ~ 9,223,372,036,854,775,807
컴퓨터가 처음 등장하고 발전하기 시작한 시점에 규정된 데이타 타입들
It maybe wasteful or sometimes not enough.
다음과 같은 상황은 에러를 발생한다.
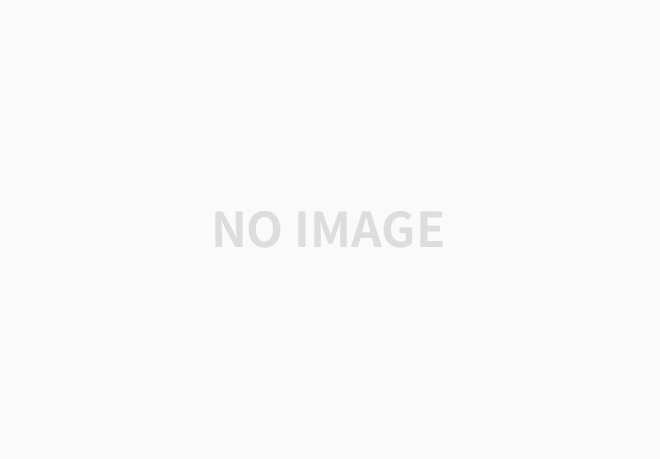
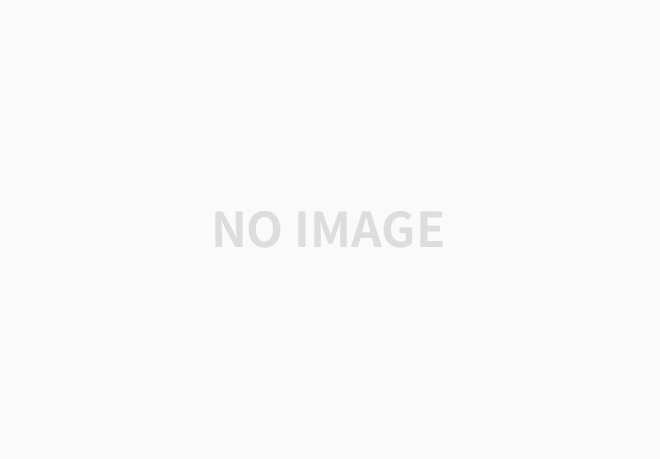
public static void main(String[] args) {
byte myByte = 127;
boolean myFlag = true;
short myBigShort =32767; //32768 => error
Actually char can hold numbers
(과거 관습적인 습관에 따라서)
char biggerShort = 64000;
그런데 print를 하면
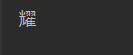
위와 같은 문자를 출력
하지만 Short보다 큰 데이터를 저장할 수 있다는 점에 주목.
Floating numbers
float과 double로 표현한다.
they store data pretty differently.
3.141592
=
0100
0000
0100
1001
0000
1111
1101
1000
여기서 0과 1이 저장되는 방식이 다르다.
think about
1/2 1/4 1/8 1/16 1/32 1/64 1/128
0.5 를 저장한다고 하면
0.1 in binary.
0.75를 저장한다고 하면
0.11 in binary
그렇다면 0.141592 => how can this be encoded in binary ?
1) we need to find the largest fractions = 1/ 8 = 0.125 => 0.001 in binary
2) 부족하므로 다음 것을 찾는다 (1/16, 1/32 too big) => 1/64 = > 0.00001 in binary
approximately
=> 0.0010010001 in binary
=> for fractions whose decimal equivalent is never ending like pi or whose decimal equivalent is so long => would exceed the bits
=> never could get exact number
=> 결국 approximate
일반적으로 ok 될 수 있지만 정확한 money or number calculation이 필요한 경우에 문제가 될 수 있다
따라서 fraction or decimal numbersp에 대해서는
=> 정확도의 개념이 등장

so float numbers를 만들때에는 f를 기입해주어야 한다.
public class NumberStuff {
public static void main(String[] args) {
byte myByte = 127;
boolean myFlag = true;
short myBigShort =32767; //32768 => error
char myChar = '강';
char myA = 'A'; // in memory 65
char biggerShort = 64000;
double myDouble = 3.141592;
float myFloat = 3.141592f;
System.out.println(myFloat);
}
}
이제 문제를 살펴보자.
public class NumberStuff {
public static void main(String[] args) {
double num1 = 2.15;
double num2 = 1.10;
System.out.println(num1 - num2);
}
}
supposed to getting 1.05
however it actually returns
1.0499999999999998
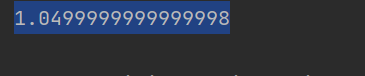
these decimal values have to be approximated when being stored in binary
more preciion이 가능할까?
double => float with specification indicator f
public class NumberStuff {
public static void main(String[] args) {
float num1 = 2.15f;
float num2 = 1.10f;
System.out.println(num1 - num2);
}
}
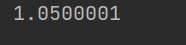
much closer
yet still receiving an approximation.
float and double은 at best approximation이라는 점이다.
16진법과 2진법의 숫자를 만들어보자.
byte anotherByte = 0x1f;
int anotherInt = 0b1010101;
why you might want to do this ?
예를 들어, 여기서 flags를 추가할 때

여기서의 함수들이 사실은 binary 계산을 근거로 한다는 것이다. (or hexadecimal)
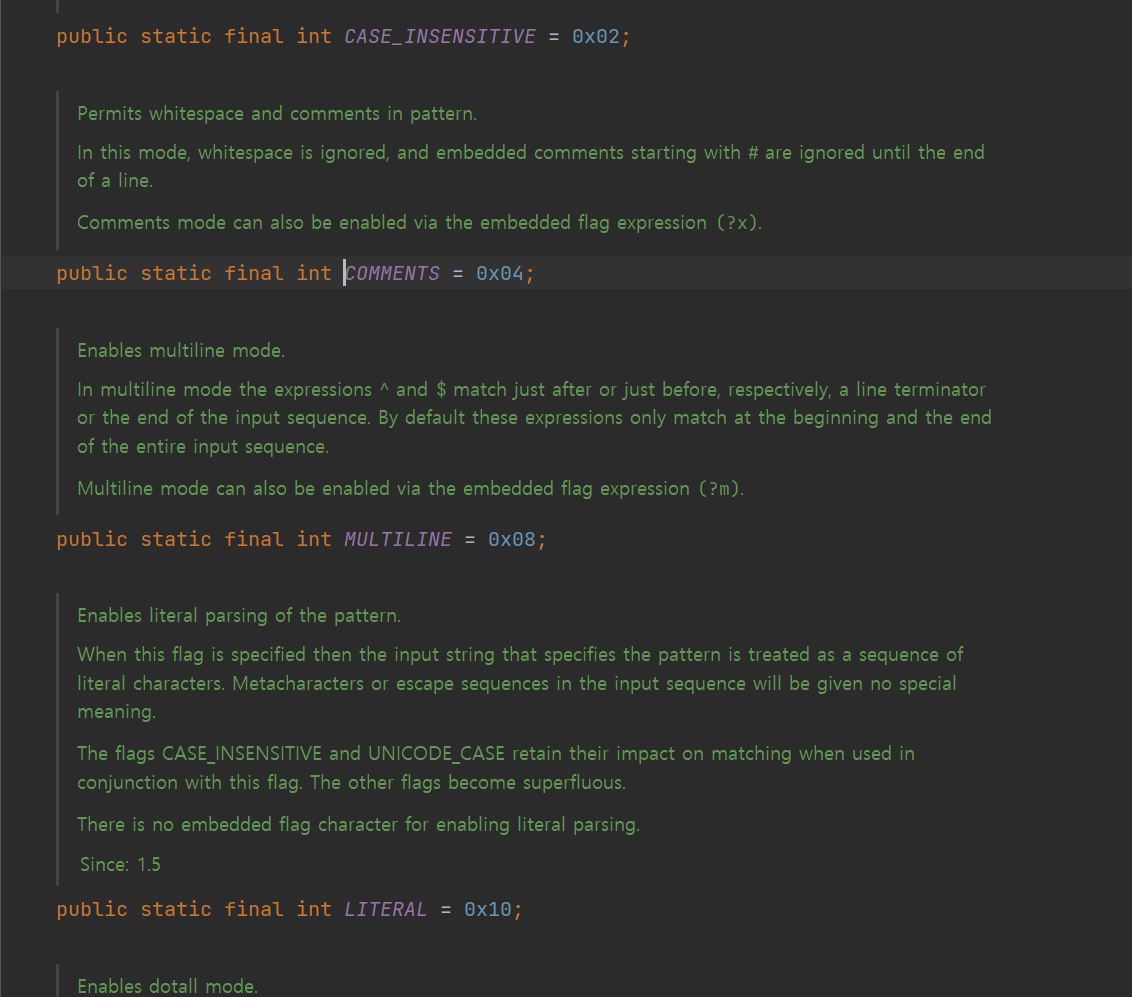
why ?
each of these represents one bit in a binaray number
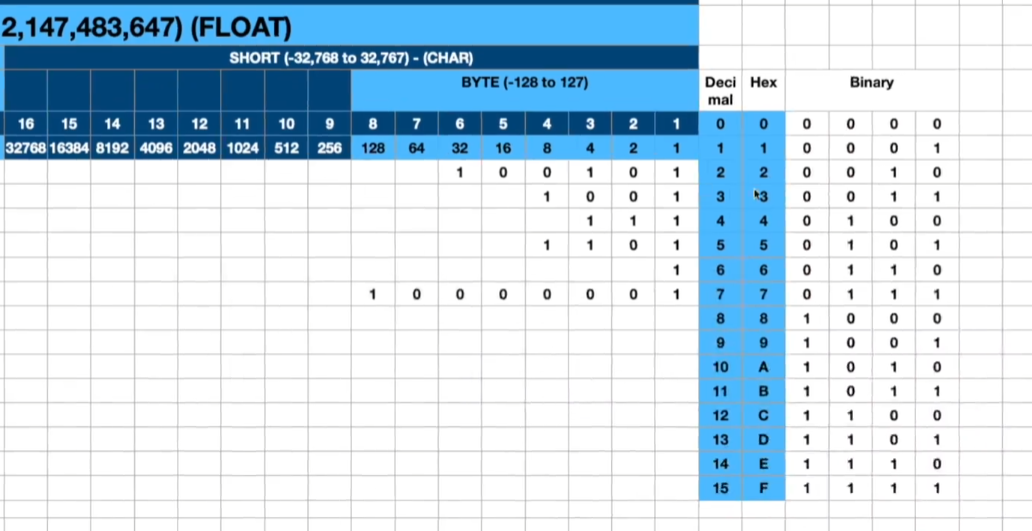
so if we were to specify that we wanted this contant here Unix lines,
that would set a one as of binary.
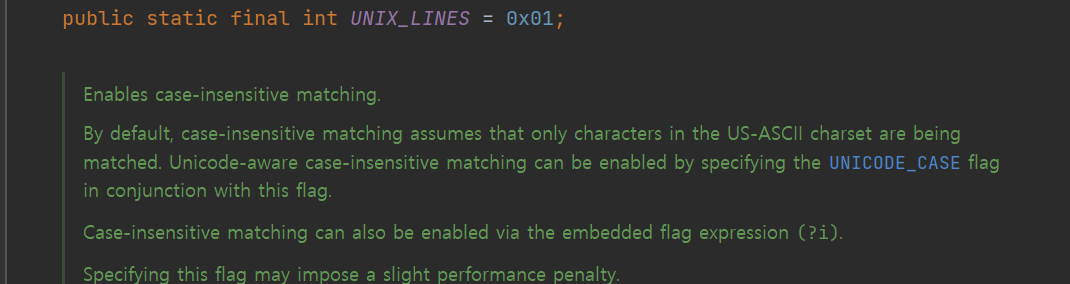
flipping toggle을 할 때 이진법 기준으로 한다는 것.
so, when you put "|", or symbol, to combine all of the different bits that is encoded as binary number.
that's the way how Java puts together and works.
이진법을 표현하는 다른 방법
3을 표현하고자 할 때 ?
int anotherInt = 0b01 | 0b10;
System.out.println(anotherInt);
returns 3
or operator가 +처럼 기능한 것
= "or + ing" = oring 연산
1) first we have binary 01
2) or
3) binanry 10 = 2
왜 이런 결과가 나올까?
각각의 계산에 대해 or 연산이 들어가기 때문에 =>
=> if either of these is one, then let the final answer be one
=> meaning :
0 1
1 0
=> 1 1
=> 따라서 3이 된다.
한 공간에 대한 각각 개별적인 연산이 들어가기 때문에 두개 다 0이면 0, 1이면 1, 0 and 1이면 > or 연산일 때는 1
10진수 계산에서도 동일한 로직이 작동
int anotherInt = 1 | 2;
System.out.println(anotherInt);
return 3
따라서
Pattern pat = Pattern.compile(regex, Pattern.COMMENTS | Pattern.DOTALL | Pattern.CANON_EQ);
이와 같은 표현은 조건을 adding up 하는 로직이 된다.
and 연산이라면 ?
only yields when both are the same and that returns 1
int anotherInt = 0b01 | 0b10 | 0b100;
System.out.println(anotherInt & 4);
=> returns 4
마지막 인자를 0으로 바꾸면 ?
int anotherInt = 0b01 | 0b10 | 0b00;
System.out.println(anotherInt & 4);
=> 0을 리턴
여기서 4가 masking을 한다고 표현할 수 있다.
네트워크에서 사용하는 ip도 binary로 표현할 수 있는데
// 5.5.5.3
// 0b0101 0b0101 0b0101 0b0011
// Netmask or Network mask
masking 되는 숫자를 사용해서 control이 가능해진다.
the way the network mask is used => any that bits correspond that is set to network mask => configure the numbers
=> NO WORRY if you don't 100 % understand this concepts.
glimps of this hints regex and raw data type works => will do when you are blocked someday